Payment Links
Payment links are the simplest way for customers to complete on-chain actions with their crypto, whether that's paying for a product, minting an NFT, or depositing funds into your app. Set which chain and token you want to accept, and let your customers pay with the tokens they already own.
In this guide we will look at how to create payment links and how to integrate payment links in your app using a hosted flow.
Payment links are intended for single use. Once a payment is completed, the link will redirect to a confirmation page or redirect to specified redirect URI.
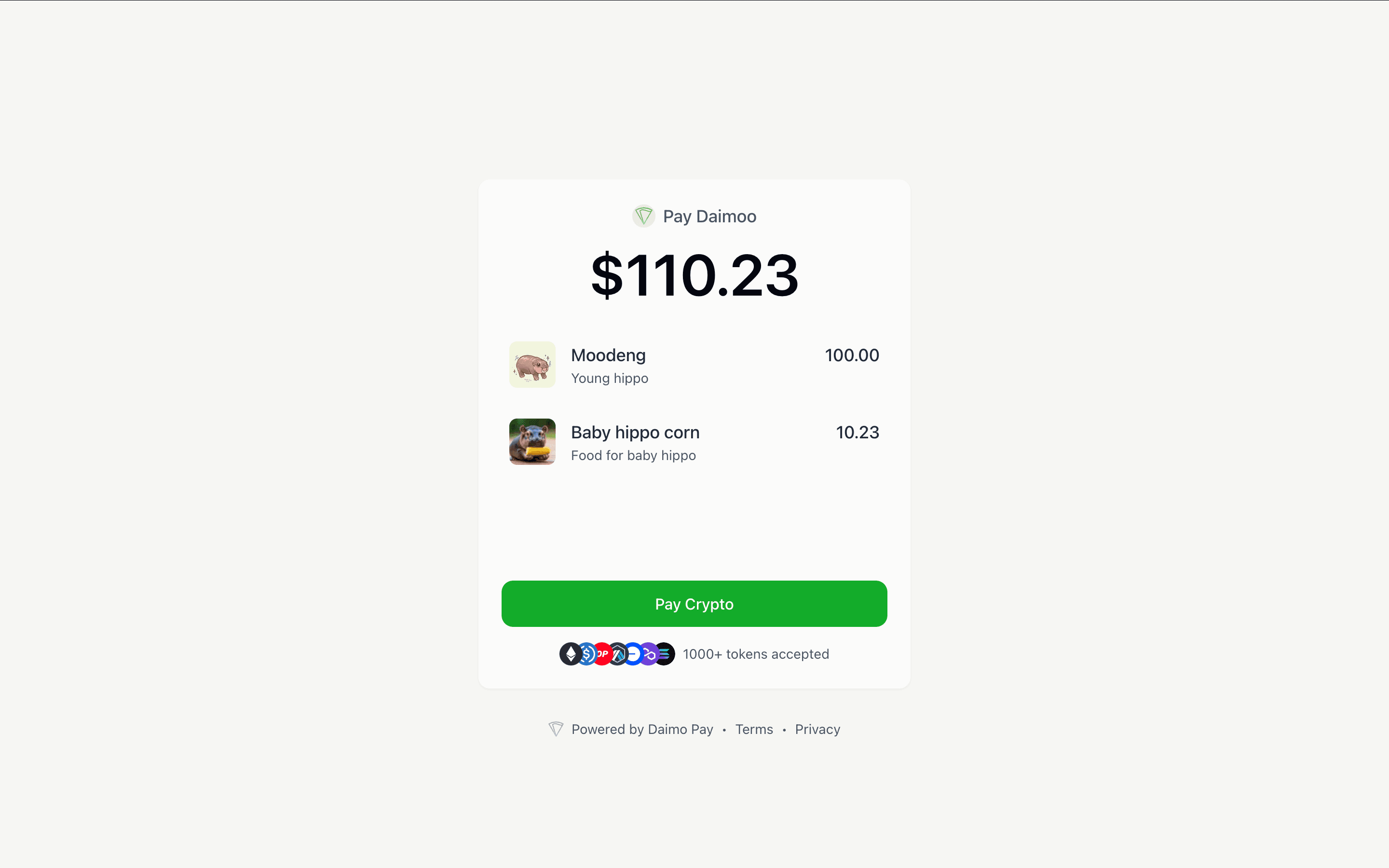
Use Cases
1. Accept crypto payment
Payment links enable you to accept crypto payments from customers in any token across multiple chains, but receive your earnings in your preferred token on your preferred chain.
For example:
- You want to receive USDC on Optimism.
- Your customer prefers to pay with a different token on a different chain.
- The customer pays with their preferred token.
- You receive USDC on Optimism within seconds.
The bridging and swapping is automatically done by Daimo, requiring no additional steps for you or your customer.
2. Cross-chain bridge and swap with a contract call
This use case is intended for developers who want to enable cross-chain and any-token support for their apps with no changes to their contracts. Instead of receiving payment with a ERC-20 or native token transfer, you can make an arbitrary contract call.
This enables use cases like:
- Splitting customer payments between multiple recipients.
- Allowing customers to pay for NFTs or digital assets with any token.
- Tracking payments with your own custom contract.
- Enabling complex dapp actions using any token on any chain.
Hosted Flow
The hosted flow is recommended for the quickest no-code integration with Daimo
Pay payment links. The https://pay.daimo.com
hosted page will allow redirected customers to:
- Connect their wallet or deposit funds from an exchange like Coinbase
- Select the token to pay with from a list of tokens they own
- Complete a transfer
On successful payment, funds will be sent to the payment recipient on the selected destination chain and token.
To get started, create a payment link using the Payments API. The response will include a url
that you can use to redirect your customers to the hosted payment page.
Customers are redirected to a payment confirmation page or a custom redirect URI after completing the payment.
// Sample response from the Payments API
{
"id": "7Tt3HUMmU83PPvd2djwcsHSZ8upMNW4sTL7a8eFQhWiQ",
"url": "https://pay.daimo.com/checkout?id=7Tt3HUMmU83PPvd2djwcsHSZ8upMNW4sTL7a8eFQhWiQ",
"payment": {
"id": "7Tt3HUMmU83PPvd2djwcsHSZ8upMNW4sTL7a8eFQhWiQ",
"status": "payment_unpaid",
"createdAt": "1739554073",
"display": {
"intent": "Pay Daimoo",
"paymentValue": "1.00",
"currency": "USD"
},
"source": null,
"destination": {
"destinationAddress": "0x3a321372E8a9755cD2CA6114eB8dA32A14F8100b",
"txHash": null,
"chainId": "8453",
"amountUnits": "1.00",
"tokenSymbol": "DAI",
"tokenAddress": "0x50c5725949A6F0c72E6C4a641F24049A917DB0Cb",
"calldata": "0x"
},
"externalId": "123",
"metadata": {
"mySystemId": "123",
"name": "John Doe"
}
}
}
Refunds
If your payment link specifies a contract call, there is a possibility that the recipient contract fails or reverts during the call. For such cases, Daimo Pay is designed to automatically attempt a refund to the customer. In most cases, the refund will be sent back directly to the customer's wallet, but in the recipient's desired currency and chain. In rare cases, it may not be possible to refund the customer's address directly (for example, if the customer pays via an exchange), in which case we will notify you to handle the refund operations manually.